本記事では、C# WPFアプリケーション ListBox でObservableCollection の指定メンバ変数だけを表示する方法を書きます。
指定メンバ変数だけを表示するには、ItemTemplate のDataTemplateを自分で設定する必要があります。
開発環境
- Windows10
- Microsoft Visual Studio Community2022
- .NET 6
- WPF アプリ
目次
設定方法
Xaml ファイルです。ListBox.ItemTemplate タグ→DataTemplateタグ→TextBlockタグ のTextタグにBindingを設定します。
ObserveableCollection の指定メンバ変数でListBox に表示するためには、ItemTemplate のDataTemplateを自分で設定する必要があります。
<Window x:Class="ListBoxProject.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ListBoxProject"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="500">
<Grid>
<ListBox x:Name="ListBox_FriutsList" VerticalAlignment="Top" HorizontalAlignment="Left" Width="200" Height="200" Margin="40,40,0,0" d:ItemsSource="{x:Null}" SelectionChanged="ListBox_SelectionChanged">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding _name}" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Window>
DataTemplateタグの子タグの設定を変えれば、ListBoxの表示項目を自由にカスタマイズできます。
Xaml と相互作用のcsファイルです。
- MainWindow コンストラクタで、リストボックスのItemsSourceにObservableCollectionを設定
- ObservableCollection で指定するFriuts クラスのメンバ変数名に_nameを含める。
using System.Collections.ObjectModel;
using System.Windows;
using System.Windows.Controls;
namespace ListBoxProject
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
private static ObservableCollection<Fruits> FruitsList = new ObservableCollection<Fruits>()
{
new Fruits( "apple", "りんご" ),
new Fruits( "banana", "ばなな" ),
new Fruits( "orange", "みかん"),
};
public MainWindow()
{
InitializeComponent();
ListBox_FriutsList.ItemsSource = FruitsList;
}
/// <summary>
/// フルーツ
/// </summary>
private class Fruits
{
public string _id { get; set; }
public string _name { get; set; }
public Fruits(string _id, string _name)
{
this._id = _id;
this._name = _name;
}
}
/// <summary>
/// アイテムクリック
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void ListBox_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
int selectedIndex = ListBox_FriutsList.SelectedIndex;
if (selectedIndex != -1)
{
return;
}
string id = FruitsList[selectedIndex]._id;
}
}
}
このように設定することで、ListBox に_nameで指定したものが表示されます。
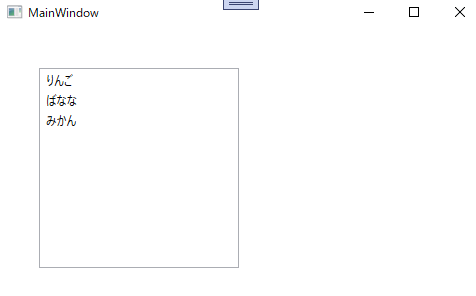
また、「ばなな」をクリックしたら、「ばなな」のid“banana” を取得することができます。
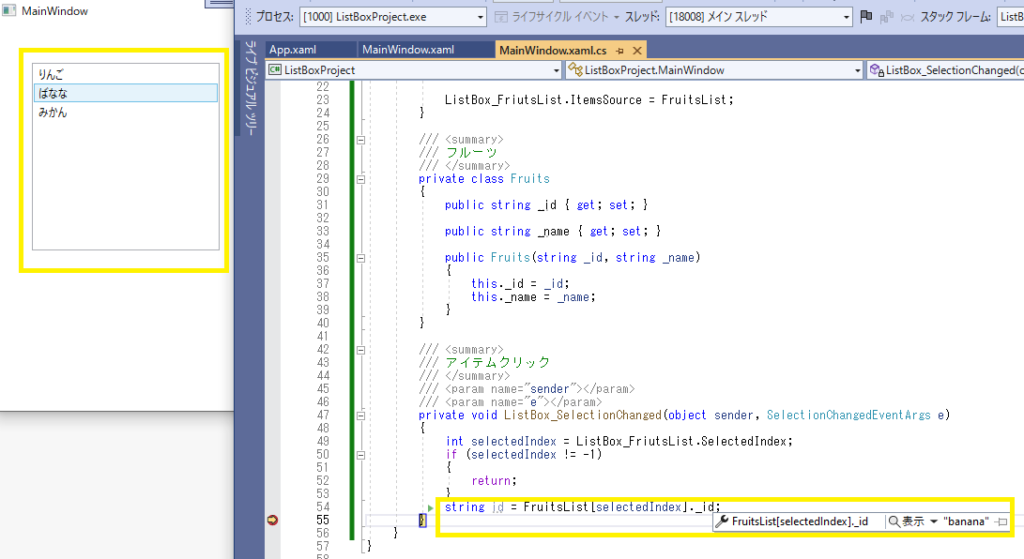
まとめ
C# WPFアプリケーション ListBox でObservableCollection で指定メンバ変数だけを表示する方法を書きます。
指定メンバ変数だけを表示するには、ItemTemplate のDataTemplateを自分で設定する必要があります。
コメント